Does Set Affect The Entire Register Assembly
x86 Assembly Guide
Contents: Registers | Retention and Addressing | Instructions | Calling Convention
This guide describes the basics of 32-bit x86 assembly language programming, roofing a small simply useful subset of the available instructions and assembler directives. There are several different assembly languages for generating x86 machine lawmaking. The one nosotros will use in CS216 is the Microsoft Macro Assembler (MASM) assembler. MASM uses the standard Intel syntax for writing x86 assembly code.
The total x86 education prepare is large and complex (Intel's x86 instruction set manuals incorporate over 2900 pages), and we do not cover it all in this guide. For example, there is a 16-bit subset of the x86 instruction set. Using the 16-bit programming model can be quite circuitous. It has a segmented retention model, more restrictions on register usage, and then on. In this guide, nosotros volition limit our attention to more modern aspects of x86 programming, and delve into the instruction set only in enough particular to go a basic feel for x86 programming.
Resources
- Guide to Using Associates in Visual Studio — a tutorial on building and debugging assembly code in Visual Studio
- Intel x86 Education Ready Reference
- Intel'southward Pentium Manuals (the full gory details)
Registers
Mod (i.e 386 and beyond) x86 processors have 8 32-bit general purpose registers, as depicted in Figure i. The register names are mostly historical. For case, EAX used to be called the accumulator since it was used past a number of arithmetics operations, and ECX was known as the counter since it was used to hold a loop alphabetize. Whereas nigh of the registers have lost their special purposes in the modern instruction set, by convention, ii are reserved for special purposes — the stack arrow (ESP) and the base pointer (EBP).
For the EAX, EBX, ECX, and EDX registers, subsections may exist used. For example, the least significant 2 bytes of EAX tin can be treated as a sixteen-bit annals called AX. The least significant byte of AX can be used as a single 8-scrap register called AL, while the most pregnant byte of AX tin can be used as a single eight-scrap register chosen AH. These names refer to the same physical register. When a ii-byte quantity is placed into DX, the update affects the value of DH, DL, and EDX. These sub-registers are mainly hold-overs from older, 16-fleck versions of the instruction set. Notwithstanding, they are sometimes user-friendly when dealing with data that are smaller than 32-bits (e.one thousand. 1-byte ASCII characters).
When referring to registers in assembly language, the names are not case-sensitive. For example, the names EAX and eax refer to the same register.
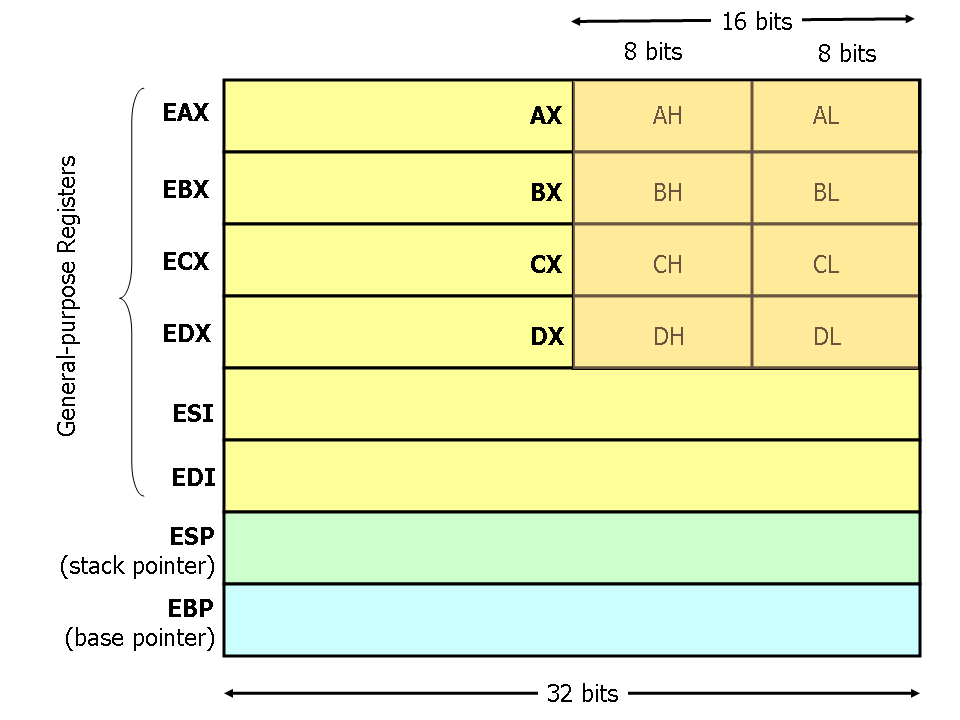
Effigy 1. x86 Registers
Memory and Addressing Modes
Declaring Static Data Regions
You can declare static information regions (analogous to global variables) in x86 assembly using special assembler directives for this purpose. Data declarations should be preceded past the .DATA directive. Following this directive, the directives DB, DW, and DD tin be used to declare one, two, and four byte data locations, respectively. Declared locations can be labeled with names for later reference — this is similar to declaring variables past name, only abides past some lower level rules. For example, locations declared in sequence will be located in retentivity next to i another.
Instance declarations:
.DATA var DB 64 ; Declare a byte, referred to every bit location var, containing the value 64. var2 DB ? ; Declare an uninitialized byte, referred to as location var2. DB 10 ; Declare a byte with no label, containing the value 10. Its location is var2 + 1. 10 DW ? ; Declare a two-byte uninitialized value, referred to as location X. Y DD 30000 ; Declare a iv-byte value, referred to as location Y, initialized to 30000.
Unlike in loftier level languages where arrays tin can accept many dimensions and are accessed by indices, arrays in x86 associates language are simply a number of cells located contiguously in memory. An array can be declared by just listing the values, every bit in the first example below. Two other common methods used for declaring arrays of information are the DUP directive and the employ of string literals. The DUP directive tells the assembler to duplicate an expression a given number of times. For example, iv DUP(2) is equivalent to 2, 2, 2, ii.
Some examples:
Z DD ane, 2, 3 ; Declare three 4-byte values, initialized to 1, 2, and 3. The value of location Z + 8 will exist 3. bytes DB ten DUP(?) ; Declare 10 uninitialized bytes starting at location bytes. arr DD 100 DUP(0) ; Declare 100 4-byte words starting at location arr, all initialized to 0 str DB 'hello',0 ; Declare 6 bytes starting at the address str, initialized to the ASCII character values for hello and the cypher (0) byte.
Addressing Memory
Modern x86-compatible processors are capable of addressing up to ii32 bytes of memory: memory addresses are 32-bits wide. In the examples above, where we used labels to refer to memory regions, these labels are actually replaced by the assembler with 32-fleck quantities that specify addresses in memory. In addition to supporting referring to memory regions past labels (i.east. constant values), the x86 provides a flexible scheme for computing and referring to memory addresses: up to two of the 32-scrap registers and a 32-chip signed constant can be added together to compute a memory accost. Ane of the registers can be optionally pre-multiplied past two, 4, or 8.
The addressing modes tin be used with many x86 instructions (we'll draw them in the next section). Here we illustrate some examples using the mov teaching that moves data between registers and retention. This educational activity has two operands: the kickoff is the destination and the second specifies the source.
Some examples of mov instructions using address computations are:
mov eax, [ebx] ; Move the 4 bytes in retentiveness at the accost contained in EBX into EAX mov [var], ebx ; Motion the contents of EBX into the four bytes at memory address var. (Note, var is a 32-bit constant). mov eax, [esi-4] ; Move four bytes at memory address ESI + (-four) into EAX mov [esi+eax], cl ; Motility the contents of CL into the byte at address ESI+EAX mov edx, [esi+iv*ebx] ; Move the 4 bytes of data at accost ESI+4*EBX into EDX
Some examples of invalid address calculations include:
mov eax, [ebx-ecx] ; Can but add annals values mov [eax+esi+edi], ebx ; At most ii registers in address ciphering
Size Directives
In full general, the intended size of the data particular at a given retentiveness accost can be inferred from the assembly code educational activity in which it is referenced. For case, in all of the above instructions, the size of the retention regions could be inferred from the size of the register operand. When we were loading a 32-scrap register, the assembler could infer that the region of retentiveness we were referring to was iv bytes broad. When nosotros were storing the value of a one byte annals to memory, the assembler could infer that nosotros wanted the accost to refer to a single byte in retentiveness.
All the same, in some cases the size of a referred-to memory region is ambiguous. Consider the instruction mov [ebx], 2. Should this instruction move the value 2 into the single byte at address EBX? Perhaps information technology should motion the 32-bit integer representation of ii into the 4-bytes starting at address EBX. Since either is a valid possible estimation, the assembler must exist explicitly directed as to which is correct. The size directives BYTE PTR, Discussion PTR, and DWORD PTR serve this purpose, indicating sizes of i, 2, and 4 bytes respectively.
For case:
mov BYTE PTR [ebx], 2 ; Move 2 into the single byte at the address stored in EBX. mov Discussion PTR [ebx], 2 ; Move the 16-chip integer representation of 2 into the 2 bytes starting at the address in EBX. mov DWORD PTR [ebx], 2 ; Move the 32-flake integer representation of ii into the 4 bytes starting at the address in EBX.
Instructions
Auto instructions by and large fall into three categories: data movement, arithmetic/logic, and control-flow. In this section, we volition look at important examples of x86 instructions from each category. This section should not be considered an exhaustive list of x86 instructions, but rather a useful subset. For a complete list, see Intel'south instruction set reference.
Nosotros apply the following annotation:
<reg32> Any 32-bit register (EAX, EBX, ECX, EDX, ESI, EDI, ESP, or EBP) <reg16> Whatever 16-bit register (AX, BX, CX, or DX) <reg8> Whatsoever viii-bit register (AH, BH, CH, DH, AL, BL, CL, or DL) <reg> Any annals <mem> A retentivity address (e.m., [eax], [var + iv], or dword ptr [eax+ebx]) <con32> Whatsoever 32-scrap constant <con16> Whatever 16-fleck constant <con8> Any 8-scrap constant <con> Whatever viii-, 16-, or 32-bit constant
Data Movement Instructions
mov — Move (Opcodes: 88, 89, 8A, 8B, 8C, 8E, ...)
The mov instruction copies the data item referred to by its second operand (i.e. register contents, memory contents, or a constant value) into the location referred to past its first operand (i.east. a register or retentivity). While register-to-annals moves are possible, direct memory-to-retentivity moves are not. In cases where retention transfers are desired, the source retention contents must kickoff be loaded into a register, then tin be stored to the destination retention address.Syntax
mov <reg>,<reg>
mov <reg>,<mem>
mov <mem>,<reg>
mov <reg>,<const>
mov <mem>,<const>
Examples
mov eax, ebx — copy the value in ebx into eax
mov byte ptr [var], 5 — store the value five into the byte at location var
button — Push stack (Opcodes: FF, 89, 8A, 8B, 8C, 8E, ...)
The push instruction places its operand onto the top of the hardware supported stack in memory. Specifically, button start decrements ESP by iv, then places its operand into the contents of the 32-bit location at address [ESP]. ESP (the stack pointer) is decremented by push since the x86 stack grows down - i.e. the stack grows from high addresses to lower addresses. Syntax
push <reg32>
button <mem>
push <con32>Examples
push eax — button eax on the stack
push [var] — button the 4 bytes at accost var onto the stack
popular — Pop stack
The pop instruction removes the four-byte data element from the height of the hardware-supported stack into the specified operand (i.east. annals or memory location). It first moves the 4 bytes located at retentiveness location [SP] into the specified register or retentivity location, and then increments SP by 4.Syntax
Examples
pop <reg32>
pop <mem>
popular edi — popular the top element of the stack into EDI.
pop [ebx] — pop the top chemical element of the stack into memory at the four bytes starting at location EBX.
lea — Load effective accost
The lea educational activity places the accost specified by its 2d operand into the annals specified past its outset operand. Note, the contents of the retentivity location are not loaded, just the constructive address is computed and placed into the register. This is useful for obtaining a pointer into a memory region.Syntax
lea <reg32>,<mem>Examples
lea edi, [ebx+4*esi] — the quantity EBX+4*ESI is placed in EDI.
lea eax, [var] — the value in var is placed in EAX.
lea eax, [val] — the value val is placed in EAX.
Arithmetics and Logic Instructions
add together — Integer Add-on
The add instruction adds together its two operands, storing the result in its first operand. Note, whereas both operands may be registers, at almost one operand may be a memory location. Syntax
add <reg>,<reg>
add together <reg>,<mem>
add <mem>,<reg>
add <reg>,<con>
add <mem>,<con>
Examples
add eax, 10 — EAX ← EAX + ten
add together BYTE PTR [var], 10 — add 10 to the single byte stored at retentivity address var
sub — Integer Subtraction
The sub teaching stores in the value of its first operand the outcome of subtracting the value of its second operand from the value of its first operand. As with add together Syntax
sub <reg>,<reg>
sub <reg>,<mem>
sub <mem>,<reg>
sub <reg>,<con>
sub <mem>,<con>
Examples
sub al, ah — AL ← AL - AHsub eax, 216 — subtract 216 from the value stored in EAX
inc, dec — Increase, Decrement
The inc pedagogy increments the contents of its operand by one. The dec education decrements the contents of its operand by one.Syntax
inc <reg>
inc <mem>
dec <reg>
december <mem>Examples
dec eax — subtract 1 from the contents of EAX.
inc DWORD PTR [var] — add together one to the 32-bit integer stored at location var
imul — Integer Multiplication
The imul instruction has ii basic formats: two-operand (first 2 syntax listings above) and iii-operand (last two syntax listings to a higher place). The ii-operand form multiplies its 2 operands together and stores the result in the showtime operand. The result (i.east. starting time) operand must be a register. The three operand form multiplies its second and third operands together and stores the result in its first operand. Again, the issue operand must exist a annals. Furthermore, the third operand is restricted to beingness a abiding value. Syntax
imul <reg32>,<reg32>
imul <reg32>,<mem>
imul <reg32>,<reg32>,<con>
imul <reg32>,<mem>,<con>Examples
imul eax, [var] — multiply the contents of EAX by the 32-bit contents of the memory location var. Shop the result in EAX.
imul esi, edi, 25 — ESI → EDI * 25
idiv — Integer Division
The idiv educational activity divides the contents of the 64 flake integer EDX:EAX (constructed by viewing EDX as the most pregnant four bytes and EAX as the least significant 4 bytes) by the specified operand value. The quotient result of the sectionalisation is stored into EAX, while the remainder is placed in EDX.Syntax
idiv <reg32>
idiv <mem>Examples
idiv ebx — divide the contents of EDX:EAX by the contents of EBX. Place the quotient in EAX and the remainder in EDX.
idiv DWORD PTR [var] — divide the contents of EDX:EAX by the 32-scrap value stored at memory location var. Place the quotient in EAX and the remainder in EDX.
and, or, xor — Bitwise logical and, or and exclusive or
These instructions perform the specified logical performance (logical bitwise and, or, and sectional or, respectively) on their operands, placing the effect in the first operand location.Syntax
and <reg>,<reg>
and <reg>,<mem>
and <mem>,<reg>
and <reg>,<con>
and <mem>,<con>
or <reg>,<reg>
or <reg>,<mem>
or <mem>,<reg>
or <reg>,<con>
or <mem>,<con>
xor <reg>,<reg>
xor <reg>,<mem>
xor <mem>,<reg>
xor <reg>,<con>
xor <mem>,<con>
Examples
and eax, 0fH — clear all but the last iv bits of EAX.
xor edx, edx — set the contents of EDX to zilch.
not — Bitwise Logical Not
Logically negates the operand contents (that is, flips all bit values in the operand).Syntax
non <reg>
not <mem>Example
non BYTE PTR [var] — negate all bits in the byte at the retentivity location var.
neg — Negate
Performs the two's complement negation of the operand contents.Syntax
neg <reg>
neg <mem>Example
neg eax — EAX → - EAX
shl, shr — Shift Left, Shift Right
These instructions shift the bits in their first operand's contents left and correct, padding the resulting empty chip positions with zeros. The shifted operand can be shifted upward to 31 places. The number of bits to shift is specified by the second operand, which can exist either an 8-bit constant or the register CL. In either case, shifts counts of greater and so 31 are performed modulo 32.Syntax
shl <reg>,<con8>
shl <mem>,<con8>
shl <reg>,<cl>
shl <mem>,<cl>shr <reg>,<con8>
shr <mem>,<con8>
shr <reg>,<cl>
shr <mem>,<cl>Examples
shl eax, ane — Multiply the value of EAX by two (if the most significant flake is 0)
shr ebx, cl — Shop in EBX the floor of result of dividing the value of EBX by 2 n wherenorth is the value in CL.
Control Menses Instructions
The x86 processor maintains an instruction arrow (IP) register that is a 32-bit value indicating the location in retentivity where the current teaching starts. Normally, it increments to point to the next instruction in memory begins later execution an instruction. The IP register cannot be manipulated directly, but is updated implicitly by provided control menstruation instructions.
We utilise the notation <label> to refer to labeled locations in the program text. Labels tin can be inserted anywhere in x86 associates lawmaking text by inbound a label proper name followed by a colon. For example,
mov esi, [ebp+8] begin: xor ecx, ecx mov eax, [esi]
The second instruction in this lawmaking fragment is labeled begin. Elsewhere in the code, nosotros can refer to the retentivity location that this instruction is located at in memory using the more user-friendly symbolic name brainstorm. This label is only a convenient way of expressing the location instead of its 32-bit value.
jmp — Spring
Transfers program command catamenia to the didactics at the memory location indicated by the operand.Syntax
jmp <label>Example
jmp begin — Bound to the educational activity labeled brainstorm.
jcondition — Provisional Jump
These instructions are conditional jumps that are based on the status of a gear up of condition codes that are stored in a special register chosen the machine status word. The contents of the auto status discussion include information about the last arithmetic performance performed. For example, one bit of this word indicates if the last result was cypher. Another indicates if the last outcome was negative. Based on these condition codes, a number of provisional jumps can exist performed. For example, the jz education performs a bound to the specified operand label if the consequence of the last arithmetic operation was aught. Otherwise, control proceeds to the next educational activity in sequence.A number of the conditional branches are given names that are intuitively based on the concluding functioning performed being a special compare instruction, cmp (run into below). For instance, conditional branches such as jle and jne are based on beginning performing a cmp functioning on the desired operands.
Syntax
je <label> (jump when equal)
jne <label> (leap when not equal)
jz <label> (jump when last outcome was cipher)
jg <label> (jump when greater than)
jge <label> (jump when greater than or equal to)
jl <label> (bound when less than)
jle <label> (jump when less than or equal to)Example
cmp eax, ebx
jle washedIf the contents of EAX are less than or equal to the contents of EBX, jump to the label done. Otherwise, continue to the next teaching.
cmp — Compare
Compare the values of the two specified operands, setting the condition codes in the machine status word appropriately. This pedagogy is equivalent to the sub educational activity, except the result of the subtraction is discarded instead of replacing the first operand.Syntax
cmp <reg>,<reg>
cmp <reg>,<mem>
cmp <mem>,<reg>
cmp <reg>,<con>Example
cmp DWORD PTR [var], 10
jeq loopIf the four bytes stored at location var are equal to the iv-byte integer constant 10, spring to the location labeled loop.
call, ret — Subroutine call and return
These instructions implement a subroutine call and return. The call instruction first pushes the current code location onto the hardware supported stack in memory (see the push instruction for details), and and then performs an unconditional jump to the code location indicated by the label operand. Unlike the elementary bound instructions, the call instruction saves the location to render to when the subroutine completes.The ret pedagogy implements a subroutine return mechanism. This didactics outset pops a code location off the hardware supported in-memory stack (see the pop instruction for details). It then performs an unconditional jump to the retrieved code location.
Syntax
telephone call <label>
ret
Calling Convention
To allow separate programmers to share code and develop libraries for apply past many programs, and to simplify the use of subroutines in general, programmers typically adopt a common calling convention. The calling convention is a protocol about how to call and render from routines. For instance, given a set of calling convention rules, a programmer need non examine the definition of a subroutine to determine how parameters should exist passed to that subroutine. Furthermore, given a set of calling convention rules, high-level language compilers tin can exist made to follow the rules, thus allowing hand-coded assembly language routines and loftier-level language routines to call one another.
In practice, many calling conventions are possible. Nosotros will apply the widely used C language calling convention. Following this convention will allow you to write assembly language subroutines that are safely callable from C (and C++) code, and will also enable you lot to call C library functions from your assembly language code.
The C calling convention is based heavily on the use of the hardware-supported stack. It is based on the push, popular, call, and ret instructions. Subroutine parameters are passed on the stack. Registers are saved on the stack, and local variables used by subroutines are placed in memory on the stack. The vast bulk of high-level procedural languages implemented on most processors have used like calling conventions.
The calling convention is broken into ii sets of rules. The first prepare of rules is employed past the caller of the subroutine, and the second gear up of rules is observed by the writer of the subroutine (the callee). It should be emphasized that mistakes in the observance of these rules quickly result in fatal program errors since the stack will be left in an inconsistent land; thus meticulous intendance should be used when implementing the phone call convention in your ain subroutines.
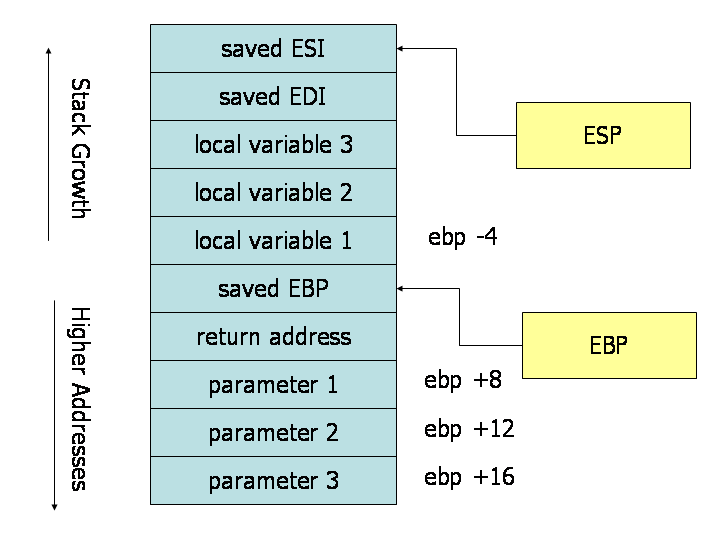
Stack during Subroutine Call
[Thanks to Maxence Faldor for providing a correct effigy and to James Peterson for finding and fixing the bug in the original version of this figure!]
A good way to visualize the functioning of the calling convention is to draw the contents of the nearby region of the stack during subroutine execution. The image in a higher place depicts the contents of the stack during the execution of a subroutine with three parameters and 3 local variables. The cells depicted in the stack are 32-bit wide retentiveness locations, thus the retentivity addresses of the cells are 4 bytes apart. The first parameter resides at an outset of viii bytes from the base of operations pointer. Above the parameters on the stack (and below the base pointer), the call education placed the return accost, thus leading to an extra iv bytes of offset from the base pointer to the first parameter. When the ret education is used to return from the subroutine, it will jump to the return address stored on the stack.
Caller Rules
To make a subrouting call, the caller should:
- Before calling a subroutine, the caller should relieve the contents of certain registers that are designated caller-saved. The caller-saved registers are EAX, ECX, EDX. Since the called subroutine is allowed to modify these registers, if the caller relies on their values afterward the subroutine returns, the caller must button the values in these registers onto the stack (then they can be restore later the subroutine returns.
- To pass parameters to the subroutine, push them onto the stack before the phone call. The parameters should be pushed in inverted order (i.e. terminal parameter start). Since the stack grows downwardly, the first parameter volition be stored at the lowest accost (this inversion of parameters was historically used to allow functions to be passed a variable number of parameters).
- To telephone call the subroutine, use the call educational activity. This didactics places the return address on acme of the parameters on the stack, and branches to the subroutine code. This invokes the subroutine, which should follow the callee rules below.
After the subroutine returns (immediately following the call teaching), the caller can expect to find the return value of the subroutine in the register EAX. To restore the car state, the caller should:
- Remove the parameters from stack. This restores the stack to its state earlier the call was performed.
- Restore the contents of caller-saved registers (EAX, ECX, EDX) by popping them off of the stack. The caller tin can assume that no other registers were modified past the subroutine.
Example
The lawmaking below shows a function telephone call that follows the caller rules. The caller is calling a function _myFunc that takes three integer parameters. Beginning parameter is in EAX, the second parameter is the abiding 216; the third parameter is in memory location var.
push [var] ; Push last parameter first push 216 ; Push the 2d parameter button eax ; Push kickoff parameter final call _myFunc ; Call the function (assume C naming) add esp, 12
Note that afterwards the telephone call returns, the caller cleans upwards the stack using the add educational activity. We have 12 bytes (3 parameters * 4 bytes each) on the stack, and the stack grows down. Thus, to become rid of the parameters, we can simply add together 12 to the stack arrow.
The result produced by _myFunc is at present available for use in the register EAX. The values of the caller-saved registers (ECX and EDX), may have been changed. If the caller uses them after the call, it would have needed to save them on the stack before the call and restore them later it.
Callee Rules
The definition of the subroutine should adhere to the post-obit rules at the beginning of the subroutine:
- Push the value of EBP onto the stack, and and so copy the value of ESP into EBP using the following instructions:
button ebp mov ebp, esp
This initial action maintains the base pointer, EBP. The base pointer is used by convention as a bespeak of reference for finding parameters and local variables on the stack. When a subroutine is executing, the base arrow holds a copy of the stack arrow value from when the subroutine started executing. Parameters and local variables will e'er be located at known, abiding offsets abroad from the base of operations arrow value. Nosotros push button the quondam base arrow value at the offset of the subroutine so that nosotros can after restore the advisable base pointer value for the caller when the subroutine returns. Call up, the caller is not expecting the subroutine to change the value of the base pointer. We so movement the stack arrow into EBP to obtain our signal of reference for accessing parameters and local variables. - Next, allocate local variables by making space on the stack. Call back, the stack grows down, so to make infinite on the top of the stack, the stack pointer should exist decremented. The amount by which the stack pointer is decremented depends on the number and size of local variables needed. For case, if iii local integers (4 bytes each) were required, the stack pointer would need to be decremented by 12 to make space for these local variables (i.eastward., sub esp, 12). As with parameters, local variables will be located at known offsets from the base arrow.
- Next, save the values of the callee-saved registers that will be used past the function. To salve registers, push them onto the stack. The callee-saved registers are EBX, EDI, and ESI (ESP and EBP will also be preserved past the calling convention, merely need not be pushed on the stack during this step).
Later on these three deportment are performed, the torso of the subroutine may proceed. When the subroutine is returns, it must follow these steps:
- Get out the return value in EAX.
- Restore the erstwhile values of any callee-saved registers (EDI and ESI) that were modified. The register contents are restored past popping them from the stack. The registers should be popped in the inverse order that they were pushed.
- Deallocate local variables. The obvious way to practice this might exist to add together the appropriate value to the stack pointer (since the infinite was allocated by subtracting the needed amount from the stack pointer). In practice, a less mistake-decumbent manner to deallocate the variables is to move the value in the base pointer into the stack pointer: mov esp, ebp. This works considering the base arrow always contains the value that the stack arrow contained immediately prior to the resource allotment of the local variables.
- Immediately earlier returning, restore the caller's base pointer value past popping EBP off the stack. Recall that the beginning matter we did on entry to the subroutine was to push the base pointer to salvage its one-time value.
- Finally, return to the caller by executing a ret instruction. This pedagogy volition find and remove the appropriate return accost from the stack.
Note that the callee'south rules autumn cleanly into two halves that are basically mirror images of ane another. The first half of the rules apply to the kickoff of the part, and are commonly said to define the prologue to the role. The latter half of the rules apply to the terminate of the function, and are thus commonly said to define the epilogue of the function.
Example
Here is an example function definition that follows the callee rules:
.486 .MODEL FLAT .CODE PUBLIC _myFunc _myFunc PROC ; Subroutine Prologue push ebp ; Save the old base pointer value. mov ebp, esp ; Set up the new base arrow value. sub esp, 4 ; Brand room for one 4-byte local variable. push edi ; Salve the values of registers that the office push esi ; will modify. This function uses EDI and ESI. ; (no need to save EBX, EBP, or ESP) ; Subroutine Body mov eax, [ebp+viii] ; Movement value of parameter one into EAX mov esi, [ebp+12] ; Motility value of parameter two into ESI mov edi, [ebp+16] ; Motion value of parameter three into EDI mov [ebp-iv], edi ; Move EDI into the local variable add [ebp-4], esi ; Add together ESI into the local variable add together eax, [ebp-4] ; Add the contents of the local variable ; into EAX (concluding result) ; Subroutine Epilogue popular esi ; Recover register values pop edi mov esp, ebp ; Deallocate local variables popular ebp ; Restore the caller's base pointer value ret _myFunc ENDP Stop
The subroutine prologue performs the standard actions of saving a snapshot of the stack pointer in EBP (the base of operations pointer), allocating local variables by decrementing the stack pointer, and saving register values on the stack.
In the body of the subroutine we can run across the utilize of the base arrow. Both parameters and local variables are located at abiding offsets from the base arrow for the duration of the subroutines execution. In particular, nosotros notice that since parameters were placed onto the stack earlier the subroutine was called, they are always located beneath the base pointer (i.e. at higher addresses) on the stack. The first parameter to the subroutine can e'er be found at memory location EBP + 8, the second at EBP + 12, the third at EBP + xvi. Similarly, since local variables are allocated afterwards the base of operations pointer is prepare, they always reside above the base arrow (i.e. at lower addresses) on the stack. In particular, the kickoff local variable is always located at EBP - 4, the second at EBP - 8, and so on. This conventional use of the base arrow allows us to quickly place the use of local variables and parameters within a function body.
The function epilogue is basically a mirror image of the role prologue. The caller'south register values are recovered from the stack, the local variables are deallocated by resetting the stack pointer, the caller'southward base of operations pointer value is recovered, and the ret instruction is used to return to the appropriate lawmaking location in the caller.
Using these Materials
These materials are released under a Creative Commons Attribution-Noncommercial-Share Alike three.0 U.s.a. License. We are delighted when people want to use or adapt the course materials nosotros developed, and yous are welcome to reuse and arrange these materials for whatever non-commercial purposes (if you would like to utilize them for a commercial purpose, please contact David Evans for more information). If you do adapt or use these materials, please include a credit like "Adapted from materials adult for University of Virginia cs216 by David Evans. This guide was revised for cs216 by David Evans, based on materials originally created by Adam Ferrari many years ago, and since updated past Alan Batson, Mike Lack, and Anita Jones." and a link back to this page.
Does Set Affect The Entire Register Assembly,
Source: https://www.cs.virginia.edu/~evans/cs216/guides/x86.html
Posted by: randallwhising.blogspot.com
0 Response to "Does Set Affect The Entire Register Assembly"
Post a Comment